Exploring Zoho Catalyst DevOps
Zoho Catalyst DevOps is a platform designed to help software teams work better together. It brings development and operations closer, making collaboration smooth.
Published on February 27, 2024
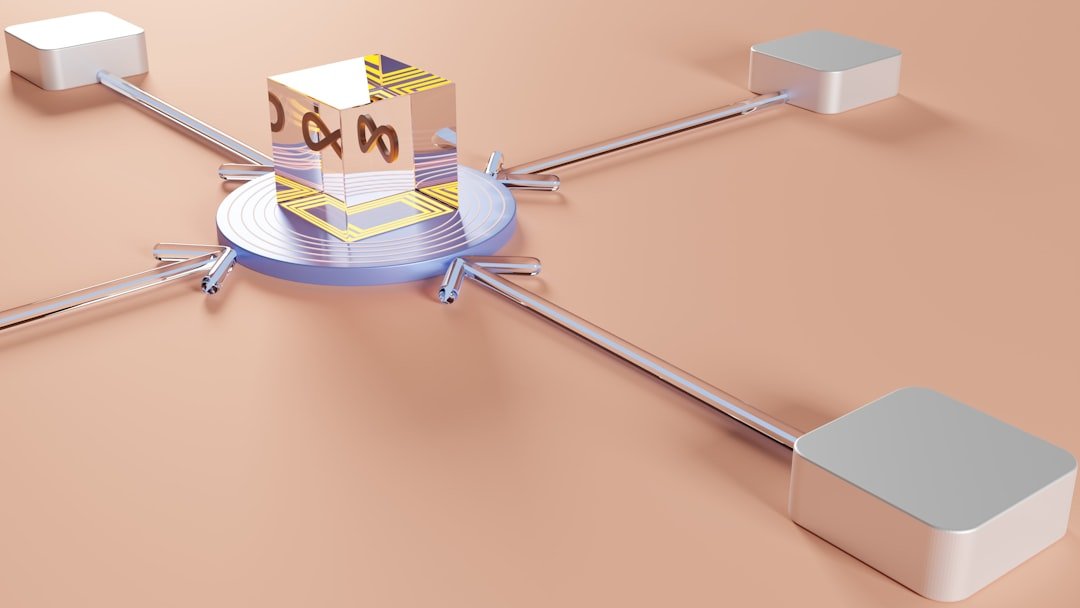
Zoho Catalyst DevOps is a platform designed to help software teams work better together. It brings development and operations closer, making collaboration smooth. It focuses on automating tasks and ensuring software delivery without issues. The platform includes a set of components that allow you to build software reliably, with solid continuous integration and deployment. In this article series, we will look at the different components, the reasons why you need them, and how they would make your software development process reliable and robust.
The functioning of the components will be shown in a sample full-stack project! So, let’s get started.
Catalyst DevOps components
In Catalyst, we have three main components that further offer more services. Let us look into them and give you a brief overview.
Monitoring
Monitoring in DevOps is a fundamental practice that involves the systematic observation and analysis of various components within a software system to ensure its optimal performance and reliability. Through continuous collection of metrics, such as resource utilization, response times, and error rates, monitoring allows DevOps teams and developers to proactively identify and address issues before they impact end-users.
Catalyst DevOps utilizes specialized tools and technologies, and teams set up alerts to notify them of deviations from normal behavior, enabling swift incident response. Effective monitoring encompasses infrastructure, application, and user-centric metrics, providing a holistic view of the system’s health. Below are the services inside the catalyst monitoring component.
Application Alerts:
Significance: Receive immediate email alerts in response to failures, timeouts, or code exceptions in specific components of your software.
Utility: Swift alerts enable prompt issue resolution, preserving application uptime and performance quality. This proactive feature mitigates potential impacts on end-users.
Logs:
Significance: Access the logs for Catalyst Serverless function execution and AppSail services.
Utility: Examination of execution details aids in error identification, facilitating issue resolution during testing phases and supporting ongoing software maintenance.
Metrics:
Significance: Monitor the resource utilization of various Catalyst components and obtain insightful reports on the application’s model.
Utility: Analysis of metrics offers actionable insights for informed decision-making. Understanding component resource usage is pivotal for optimizing the project area and hence increasing the revenue.
Application Performance Monitoring (APM):
Significance: APM delivers comprehensive reports on Catalyst Serverless function executions. This is a level up from the basic logging service provided.
Utility: By providing detailed statistics on functional invocations, errors, and response times, APM aids in swiftly identifying, analyzing, and addressing performance bottlenecks.
Quality
To ensure that users are provided with robust and fail-proof software, we have to make sure that the DevOps pipelines cater to the required protocols and tools. It is an integral part of the software development and delivery lifecycle. The pipeline ensures that the code is thoroughly tested and validated from time to time. Automated testing plays a central role which takes a huge testing load from the QA team.
In Catalyst DevOps, Automation testing is the main module that ensures your APIs are end-to-end tested.
Automated Testing
Significance: Automated testing makes your endpoints error-free and keeps you aware of potential pitfalls.
Utility: You get test cases according to your requirements with multiple test cases under a single test suite. Scheduling of test cases can also be configured for total automation.
Repositories
In DevOps, repositories serve as centralized locations for storing and managing version-controlled code, configurations, and other artifacts that make up an application or system. Version control systems, such as Git, are commonly used to facilitate collaborative development, enabling multiple team members to work concurrently on the same codebase. Repositories play a critical role in supporting Continuous Integration (CI) and Continuous Deployment (CD) practices by providing a structured and organized environment for code changes.
Developers commit changes to the repository, triggering automated build and testing processes that help maintain code quality. Branching and merging within repositories allow for parallel development efforts and the integration of new features. Catalyst supports Github repositories and allows complete synchronization between the platforms. You can work on Git Hub and deploy your repo on the catalyst account.
GitHub Integration
Significance: GitHub integration in Zoho DevOps is pivotal for streamlined collaboration, version control, and efficient code management.
Utility: This integration allows seamless synchronization with GitHub repositories, facilitating a smooth workflow for developers. It ensures version-controlled code, simplified collaboration, and an organized environment for code changes. Developers can benefit from a cohesive and well-coordinated development process, enhancing overall productivity and code quality.
Let’s get practical
Now that we are aware of the components that are included in Zoho DevOps, let us create a project that will demonstrate the use of the components. For this purpose, we will be creating an expense-tracking application with frontend and catalyst serverless functions. Without any delay, let’s get started!
Prerequisites
Before we start the development, you should have the following things installed and set up on your local machine.
– A Zoho account
– Zoho catalyst cli installed globally on your machine.
– An IDE for coding.
– Nodejs installed
Once you have these, write the following command and initialize a new project.
catalyst init
- Choose client and functions for the project.
- For functions, we will be going with advanced IO.
- For client, you can choose react, angular or basic javascript; anything you feel comfortable in. For the project, we will be going with basic javascript.
Frontend
With the basic web app, we the the following app directory
Let us add code in each of the files for our expense tracker.
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Expense Tracker</title>
<link rel="stylesheet" href="main.css" />
</head>
<body>
<div id="container">
<div id="app">
<h1>Expense Tracker</h1>
<div id="addExpenseForm">
<input type="text" id="description" placeholder="Description" />
<input type="number" id="amount" placeholder="Amount" />
<button onclick="addExpense()">Add Expense</button>
</div>
<table id="expenses">
<thead>
<tr>
<th>Description</th>
<th>Amount</th>
</tr>
</thead>
<tbody id="expenseList"></tbody>
</table>
</div>
</div>
<script src="main.js"></script>
</body>
</html>
Main.css
#container {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #f4f4f4;
}
#app {
text-align: center;
}
#expenses {
margin-top: 20px;
border-collapse: collapse;
width: 100%;
}
#expenses th,
#expenses td {
padding: 10px;
border: 1px solid #ddd;
}
#addExpenseForm {
margin-top: 20px;
display: flex;
flex-direction: column;
max-width: 300px;
margin-left: auto;
margin-right: auto;
}
#addExpenseForm input,
#addExpenseForm button {
margin-bottom: 10px;
padding: 8px;
}
Main.js
const expenseList = document.getElementById("expenseList");
const descriptionInput = document.getElementById("description");
const amountInput = document.getElementById("amount");
// Function to fetch and display expenses
async function fetchExpenses() {
try {
const response = await fetch("/server/expense_tracker_function/expenses");
const data = await response.json();
// Clear existing expenses
expenseList.innerHTML = "";
if (data.length === 0) {
const newRow = document.createElement("tr");
newRow.innerHTML = `
<td colspan="2">No expenses yet</td>
`;
expenseList.appendChild(newRow);
}
// Display new expenses
data.forEach((expense) => {
const newRow = document.createElement("tr");
newRow.innerHTML = `
<td>${expense.description}</td>
<td>${expense.amount.toFixed(2)}</td>
`;
expenseList.appendChild(newRow);
});
} catch (error) {
console.error("Error fetching expenses:", error);
}
}
// Function to add a new expense
async function addExpense() {
const description = descriptionInput.value.trim();
const amount = parseFloat(amountInput.value);
if (description && !isNaN(amount)) {
try {
const response = await fetch(
"/server/expense_tracker_function/expenses",
{
method: "POST",
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({ description, amount }),
}
);
if (response.ok) {
// Fetch and display updated expenses after adding a new one
fetchExpenses();
// Clear input fields
descriptionInput.value = "";
amountInput.value = "";
} else {
console.error("Failed to add expense:", response.statusText);
}
} catch (error) {
console.error("Error adding expense:", error);
}
} else {
alert("Please enter valid description and amount.");
}
}
// Fetch and display initial expenses on page load
fetchExpenses();
The code is pretty simple and self-explanatory. We are just calling our serverless function from it and displaying the results.
Now, let us create serverless functions that will handle calls from the frontend.
Serverless function
Since we are using advanced IO, let us create an express serverless function to keep things simple yet powerful.
Go to the directory of the function and install express by using the following command.
npm install express --save
Now, add the following code in the index.js file.
const express = require("express");
const app = express();
const port = 3000;
// Parse incoming JSON requests
app.use(express.json());
// In-memory storage for simplicity (replace with a database in a real application)
let expenses = [];
// Get all expenses
app.get("/expenses", (req, res) => {
res.json(expenses);
});
// Add a new expense
app.post("/expenses", (req, res) => {
const { description, amount } = req.body;
if (description && !isNaN(amount)) {
const newExpense = {
description: description,
amount: parseFloat(amount.toFixed(2)),
};
expenses.push(newExpense);
res.status(201).json(newExpense);
} else {
res.status(400).json({ error: "Invalid data" });
}
});
// Start the server
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
module.exports = app;
Finally, time to deploy our project and see how it looks!
Run the following command to deploy the project.
catalyst deploy
If you get the following results, congrats your expense tracker is online!
Now that our application is ready, in the next part, we will be integrating Catalyst DevOps components into it!